Hello, I was trying to show events from my db but nothing is displayed, I attach the code:
Backend
<?php
require_once '_db.php';
$stmt = $db->prepare("SELECT * FROM index /*WHERE NOT ((end_datetime <= :start_datetime) OR (start_datetime >= :end_datetime))*/");
//$stmt->bindParam(':start_datetime', $_GET['start_datetime']);
//$stmt->bindParam(':end_datetime', $_GET['end_datetime']);
//$stmt->execute();
$result = $stmt->fetchAll();
class Event {}
$events = array();
foreach($result as $row) {
$e = new Event();
$e->id = $row['id'];
$e->type = $row['type'];
$e->start_datetime = $row['start_datetime'];
$e->end_datetime = $row['end_datetime'];
//$e->color = $row['color'];
//$e->resource = $row['resource_id'];
$events[] = $e;
}
header('Content-Type: application/json');
echo json_encode($events);
?>
Index
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>JavaScript Resource Calendar Tutorial (PHP/MySQL)</title>
<style>
p, body, td { font-family: Tahoma, Arial, Helvetica, sans-serif; font-size: 10pt; }
body { padding: 0px; margin: 0px; background-color: #ffffff; }
a { color: #1155a3; }
.space { margin: 10px 0px 10px 0px; }
.header { background: #003267; background: linear-gradient(to right, #011329 0%, #00639e 44%, #011329 100%); padding: 20px 10px; color: white; box-shadow: 0px 0px 10px 5px rgba(0, 0, 0, 0.75); }
.header a { color: white; }
.header h1 a { text-decoration: none; }
.header h1 { padding: 0px; margin: 0px; }
.main { padding: 10px; margin-top: 10px; }
</style>
<!-- DayPilot library -->
<script src="js/daypilot/daypilot-all.min.js"></script>
</head>
<body>
<div class="header">
<h1><a href='https://code.daypilot.org/87709/javascript-resource-calendar-tutorial-php-mysql'>JavaScript Resource Calendar Tutorial (PHP/MySQL)</a></h1>
<div><a href="https://javascript.daypilot.org/">DayPilot for JavaScript</a> - HTML5 Calendar/Scheduling Components for JavaScript/Angular/React/Vue</div>
</div>
<div class="main">
<div id="dp"></div>
</div>
<script>
const dp = new DayPilot.Calendar("dp", {
viewType: "Resources",
onTimeRangeSelected: async (args) => {
const modal = await DayPilot.Modal.prompt("Create a new event:", "Event 1");
dp.clearSelection();
if (modal.canceled) {
return;
}
const params = {
start: args.start,
end: args.end,
text: modal.result,
resource: args.resource
};
const {data} = await DayPilot.Http.post("backend_create.php", params);
params.id = data.id;
dp.events.add(params);
},
onEventMoved: async (args) => {
const params = {
id: args.e.id(),
start: args.newStart,
end: args.newEnd,
resource: args.newResource
};
await DayPilot.Http.post("backend_move.php", params);
},
onEventResized: async (args) => {
const params = {
id: args.e.id(),
start: args.newStart,
end: args.newEnd,
resource: args.newResource
};
await DayPilot.Http.post("backend_move.php", params);
},
onEventClick: async (args) => {
const colors = [
{name: "Blue", id: "#3c78d8"},
{name: "Green", id: "#6aa84f"},
{name: "Yellow", id: "#f1c232"},
{name: "Red", id: "#cc0000"},
];
const form = [
{name: "Name", id: "text"},
{name: "Color", id: "color", type: "select", options: colors}
];
const modal = await DayPilot.Modal.form(form, args.e.data);
if (modal.canceled) {
return;
}
const data = {
id: args.e.id(),
text: modal.result.text,
color: modal.result.color
};
await DayPilot.Http.post(`backend_update.php`, data);
dp.events.update({
...args.e.data,
text: modal.result.text,
color: modal.result.color
});
console.log("Updated.");
},
onBeforeEventRender: args => {
args.data.barColor = args.data.color;
}
});
dp.init();
dp.columns.load("backend_resources.php", function success() {
// wait for columns to load
dp.events.load("backend_events.php");
});
</script>
</body>
</html>
Database:
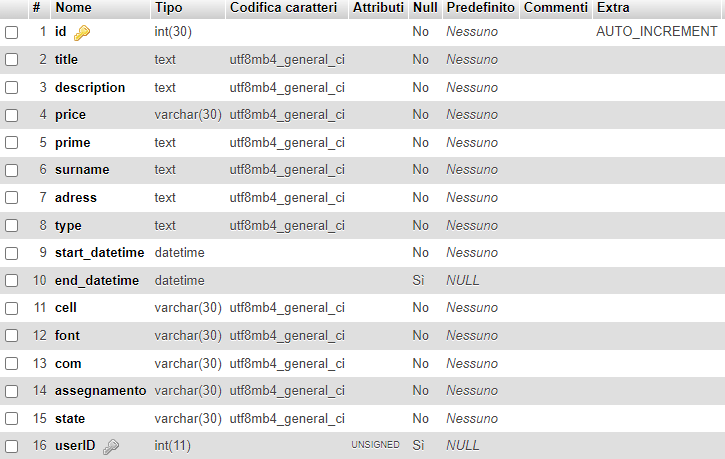